We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
用两个栈实现一个队列。队列的声明如下,请实现它的两个函数 appendTail 和 deleteHead ,分别完成在队列尾部插入整数和在队列头部删除整数的功能。(若队列中没有元素,deleteHead 操作返回 -1 )
示例 1:
输入: ["CQueue","appendTail","deleteHead","deleteHead"] [[],[3],[],[]] 输出:[null,null,3,-1]
示例 2:
输入: ["CQueue","deleteHead","appendTail","appendTail","deleteHead","deleteHead"] [[],[],[5][2],[],[]] 输出:[null,-1,null,null,5,2]
解法一:
设置一个输入栈和一个输出栈。 向队列加入数据时,将数据存入输入栈即可。 取队首元素时,先将数据存入输出栈,取出队首后,再将输出栈的数据存回输入栈。代码如下:
class CQueue { public: stack<int> in; stack<int> out; CQueue() { } void appendTail(int value) { in.push(value); } int deleteHead() { if (in.empty()) { return -1; } while (!in.empty()) { int tmp = in.top(); in.pop(); out.push(tmp); } int res = out.top(); out.pop(); while (!out.empty()) { int tmp = out.top(); out.pop(); in.push(tmp); } return res; } };
解法二:
解法一时间复杂度偏高,因为有输出栈向输入栈存回数据的操作,有优化的余地: 输出栈的元素不必存回输入栈,出队时,先检查输出栈,若有元素,则该元素即为当前的队首元素;否则,将输入栈的元素转入输出栈。如下图所示:
代码如下:
class CQueue { public: stack<int> in; stack<int> out; CQueue() { } void appendTail(int value) { in.push(value); } int deleteHead() { if (!out.empty()) { int tmp = out.top(); out.pop(); return tmp; } if (in.empty()) { return -1; } while (!in.empty()) { int tmp = in.top(); in.pop(); out.push(tmp); } int res = out.top(); out.pop(); return res; } };
Refer
The text was updated successfully, but these errors were encountered:
No branches or pull requests
用两个栈实现一个队列。队列的声明如下,请实现它的两个函数 appendTail 和 deleteHead ,分别完成在队列尾部插入整数和在队列头部删除整数的功能。(若队列中没有元素,deleteHead 操作返回 -1 )
示例 1:
示例 2:
解法一:
设置一个输入栈和一个输出栈。
向队列加入数据时,将数据存入输入栈即可。
取队首元素时,先将数据存入输出栈,取出队首后,再将输出栈的数据存回输入栈。代码如下:
解法二:
解法一时间复杂度偏高,因为有输出栈向输入栈存回数据的操作,有优化的余地:
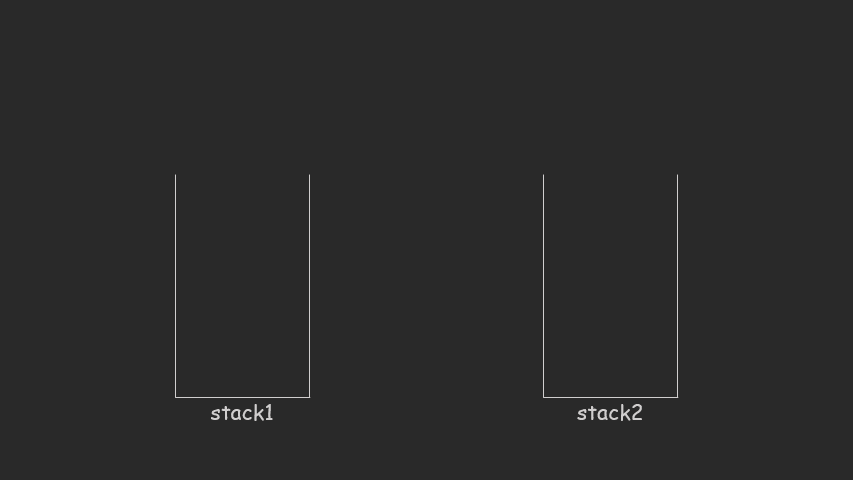
输出栈的元素不必存回输入栈,出队时,先检查输出栈,若有元素,则该元素即为当前的队首元素;否则,将输入栈的元素转入输出栈。如下图所示:
代码如下:
Refer
The text was updated successfully, but these errors were encountered: