feat: finalize param inputs + multiple fixes #82
Merged
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
Fixes #74
Fixes #75
Fixes #76
Fixes #81
Testing
Deployed the following sample:
Deployment successful:
Logs when a file is uploaded to storage;
A string param with ResourceInput to select a storage bucket, the default is set to the builtin default param params.STORAGE_BUCKET and you can see the input prompts with this value pre selected:
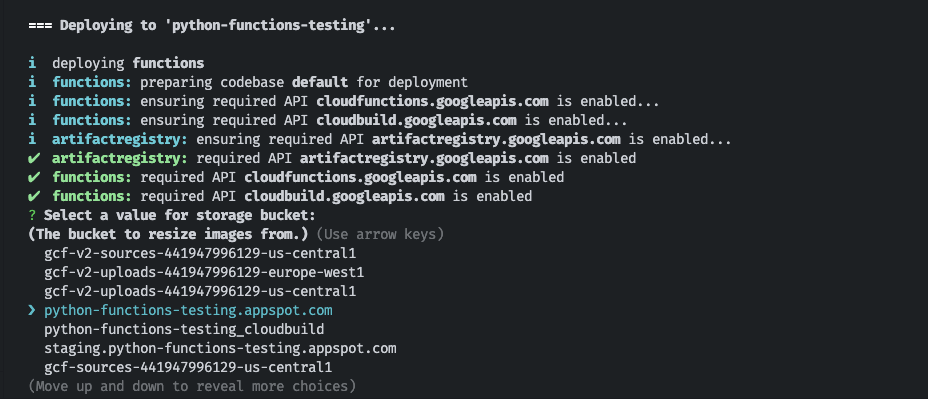
A string param with text input to select a path:

and tests regex validation:
A list param with multi select + default selection correctly showing:
A bool param with select input (also shows correct default value):
A secret param:
(shows #76 is now fixed and secrets are being created correctly now)
The CLI generates a .env file:
The generated manifest yaml: